Data Structures
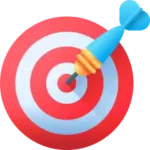
Data Structures and Algorithms Essentials
Master core computer science concepts by mastering data structures, algorithms & problem solving using C++
⭐⭐⭐⭐⭐ 4.8/5
What you'll learn
- Core concepts & internals of Data Structures
- Trees, Linked Lists, Heaps, Graphs
- Build all data structures from scratch
- Object Oriented Programming Basics
- Learn to apply Data Structures
- Brute Force & Optimisation Techniques
- Space Time Complexity Analysis
- Hash Functions, Collision Handling
- Recursion & Backtracking
- Dynamic Memory & Pointers
- Create your own DS library!
- C++ Standard Template Library Basics
- Project – Design & Implement Shopping Cart
Course Overview
Are you a beginner looking to enter the world of Data Structures or intermediate programmer wondering what happens behind a Hash-table?
Welcome to Data Structures & Algorithms, Essentials Course – the only course you need to understand the core concepts behind Data Structures & build a solid programming foundations using C++ . The course is taught by an expert instructor Praveen Kumar from Google, who is not just a software engineer but also has mentored thousands of students in becoming great programmers & developers and is top rated on Udemy for his amazing teaching skills.
Every software application revolves around data, performing different operations like Insert, Delete, Update & Search. To be a great software developer, understanding of Data Structures & Algorithms is must and this course provides you a deep understanding of the topic by covering both the theory and hands-on-implementation of each data structure from scratch.
The Course contains 20+ hours of interactive video content & dozens of coding exercises, teaching you all essential concepts starting from ground zero. Each section covers data structure in great detail, with Coding Exercises & real life examples.
Here is what you will learn :
- Bit masking
- Object Oriented Programming Basics
- Pointers & Dynamic Memory (C++)
- Recursion
- Array, 2D Array, Strings, Vectors
- Linked Lists, Stacks, Queues
- Trees, BST, Tries
- Heaps/Priority Queues
- Hash-tables, Collision Handling
- Graphs
- Brute Force, Backtracking
- Sorting & Searching
- Divide & Conquer
- Dynamic Programming
The course is designed for beginner & intermediate programmers. We try to make not so easy topics look easy with intuitive explanations & interactive video lectures with dozens of memes 😉 The course finishes with a final mini project – a command line app for an online shopping cart combining principles from Object Oriented Programming & Data Structures.
Meet the Instructor
Praveen is a popular programming instructor and an ace software engineer, currently working with Scaler and created Coding Minutes. He is known for his amazingly simplified explanations that make everyone fall in love with programming.
He has over 5+ years of teaching experience and has trained over 75,000 students in classroom boot camps & online courses in the past.